Cloudinary CDN configuration
Cloudinary support requires adding the AdaptiveImages.Cloudinary NuGet package.
Once the CDN service has been set up for the website, enabling it is as simple as:
using AdaptiveImages.Cloudinary;
using AdaptiveImages.Initialization;
using Microsoft.Extensions.DependencyInjection;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddCms()
.AddAdaptiveImages()
.AddCloudinary(new CloudinarySettings
{
CloudName = "{your Cloudinary cloud name}"
});
}
}
Separating images for different environments
By default, all transformed images are stored in the same CDN location. This can lead to unnecessary usage, as purging images used only for development and testing becomes more difficult.
To mitigate this, you may opt to set a folder prefix:
public void ConfigureServices(IServiceCollection services)
{
services.AddCms()
.AddAdaptiveImages()
.AddCloudinary(new CloudinarySettings
{
CloudName = "{your Cloudinary cloud name}",
FolderPrefix = "staging"
});
}
Note: Each folder prefix must be mapped in the CDN service.
Disable during local development
To eliminate the need for a reverse proxy (see CDN optimization and delivery), you may choose to only enable the CDN for non-development environments.
using AdaptiveImages.Cloudinary;
using AdaptiveImages.Initialization;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
public class Startup
{
private readonly IWebHostEnvironment _webHostingEnvironment;
public Startup(IWebHostEnvironment webHostingEnvironment)
{
_webHostingEnvironment = webHostingEnvironment;
}
public void ConfigureServices(IServiceCollection services)
{
services.AddCms()
.AddAdaptiveImages();
if (!_webHostingEnvironment.IsDevelopment())
{
services.AddCloudinary(new CloudinarySettings
{
CloudName = "{your Cloudinary cloud name}"
});
}
}
}
Use with your own Cloudinary account
Note: Please refer to your order confirmation for configuration details if your Adaptive Images license comes bundled with CDN service.
Customizing Cloudinary settings
services.AddCloudinary(new CloudinarySettings
{
CloudName = "your-cloud-name",
FolderPrefix = "staging",
DefaultParameters = "f_auto,dpr_auto",
PreserveTransparency = true,
HostName = "res.cloudinary.com",
MaxFilesizeToCloudinary = 10240,
MaxDimensions = 25,
LowerCaseUrls = false
});
Note: Except for
CloudName
andFolderPrefix
, the values above reflect the defaults of theCloudinarySettings
class and do not need to be set unless different from the defaults.
Configuring your Cloudinary account
Note: If you have a bundled CDN service with your Adaptive Images license, this configuration will be done automatically.
Verify your cloud name
Create a Cloudinary account if you don't have one already: https://cloudinary.com/
Sign in to Cloudinary and make note of your cloud name:
Add upload mappings
At a minimum, you need a mapping for the default image provider called optimizely (used for CMS images).
Go to Settings
Click the Upload tab
Add upload mappings as required
Folder should be set to the name of the image provider, e.g. optimizely, optionally prefixed by a folder prefix, e.g. staging.
URL prefix should be set to an accessible host name, followed by /adaptiveimages/{provider name}/
, in this case /adaptiveimages/optimizely/.
Note: The URL prefix must have a trailing slash.
Example of mappings for the optimizely image provider, with images in the staging environment being separated from production through a folder prefix called staging:
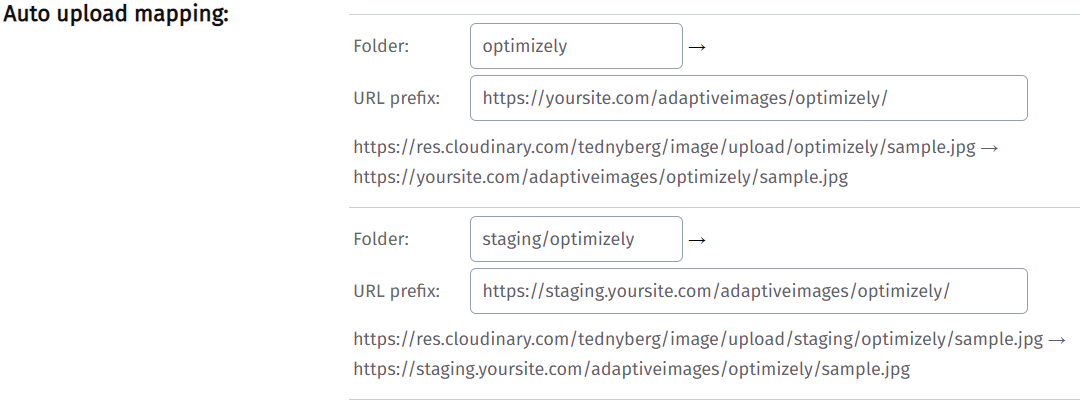
Note: You need one mapping for each image provider. If you are using folder prefixes, you need to add a mapping for each folder prefix/image provider combination.
Websites upgraded from Episerver CMS 11
If your Adaptive Images-enabled website has been upgraded from Episerver CMS 11, you may have image properties referencing the Episerver image provider.
If so, you have two options:
Add CDN upload mapping(s) for the image provider called episerver
Iterate over all
SingleImage
properties and the form factors of eachAdaptiveImage
property and change theProvider
name from episerver to optimizely